JSONAdditionalProperties
Specify a record that can have properties whose names are not listed in the definition.
Syntax
JSONAdditionalProperties = {
true |
false }
JSONAdditionalProperties
is an optional attribute. Default value is true.
Usage
You can set JSONAdditionalProperties
on a record, on a dictionary type, or a
user-defined type to specify that it can or can not
have properties in addition to those listed in the definition. For example, if you have complex data
or data that is flexible in how it may be represented, then you can specify
JSONAdditionalProperties
to validate additional properties added to the schema at
runtime.
This attribute represents the additionalProperties
keyword in the JSON schema
property of the Swagger and OpenAPI specification. By default, a JSON object accepts additional
properties. For more information on properties and additional properties, go to the JSON Schema (external link)
object page and search for additional properties.
Example 1: using JSONAdditionalProperties
JSONAdditionalProperties
attribute is set on various input
records. When the attribute has no value (neither true or false), it defaults to
"true".TYPE MyRecord RECORD ATTRIBUTE(JSONAdditionalProperties="false")
a,b INTEGER
END RECORD
TYPE MyArray DYNAMIC ARRAY OF RECORD ATTRIBUTE(JSONAdditionalProperties = "true")
a,b INTEGER
END RECORD
TYPE MyComplexType RECORD ATTRIBUTE(JSONAdditionalProperties)
a,b INTEGER
END RECORD
In the OpenAPI documentation, the GWS engine exposes the additionalProperties
property in the schema
for the record defined with
JSONAdditionalProperties
. In the following sample schema, a request using the
MyRecord
schema can not send additional properties, whereas a request using
MyComplexType
accepts additional properties. Open curly braces
({}
) denote true.
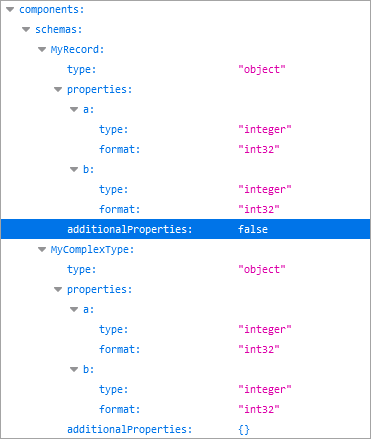
Example 2: storing additional properties
JSONAdditionalProperties
attribute is set to
store the additional properties. Therefore, you must define types in the following way: - You must declare the type itself with the
JSONAdditionalProperties
attribute. - You must also declare a dictionary type at the root of the type with the
JSONAdditionalProperties
attribute (set to "true" or without value) to store the additional properties and values.
INTEGER
value can not be returned if a STRING
is expected; if this
occurs, the serializer will return a serialization error.
TYPE MyStoredTypeString RECORD ATTRIBUTE(JSONAdditionalProperties)
a,b INTEGER,
p DICTIONARY ATTRIBUTE(JSONAdditionalProperties) OF STRING
END RECORD
TYPE MyStoredTypeRecord RECORD ATTRIBUTE(JSONAdditionalProperties)
a,b STRING,
p DICTIONARY ATTRIBUTE(JSONAdditionalProperties) OF RECORD
d,e INTEGER
END RECORD
END RECORD
Example 3: using JSONOneOf with JSONAdditionalProperties
In this example, the JSONAdditionalProperties
attribute is used with the
JSONOneOf
attribute to
validate the schemas in a record that can have one valid schema. In the oneOfType
record there are three sub-records. Each sub-record is also set with the
JSONAdditionalProperties
attribute so that each one can be considered for
validation depending on the request.
JSON schema defaults to allowing additional properties – properties that do not
correspond to any property name – which has the effect of allowing schemas to be validated with any
properties. Therefore, in our example (with JSONOneOf
alone) no schema could be
validated, since they would all be valid.
With JSONAdditionalProperties
set to false, the validation process for
oneOf
can succeed. JSON schema checks that a valid object has been received;
otherwise, the schema will be invalid and will be eliminated. In the end, according to the
oneOf
requirement, there must remain only one valid schema in all the possible
schemas.
The JSONSelector attribute specifies which sub-record to
use as set in the request from the client application. In the echoOneOfType
function, the CASE
statement tests for the _selector
value.
# The attribute JSONAdditionalProperties=false is mandatory
# Otherwise all schemas will be valid and oneof can only accept one valid schema
TYPE oneOfType RECORD ATTRIBUTE(JSONOneOf)
_selector INTEGER ATTRIBUTE(JSONSelector),
accountById RECORD ATTRIBUTE(JSONAdditionalProperties="false")
id INTEGER,
birthday DATE,
gender STRING
END RECORD,
accountByname RECORD ATTRIBUTE(JSONAdditionalProperties="false")
name STRING,
birthday DATE,
gender STRING
END RECORD,
accountBydate RECORD ATTRIBUTE(JSONAdditionalProperties="false")
date DATETIME YEAR TO SECOND,
birthday DATE,
gender STRING
END RECORD
END RECORD
FUNCTION echoOneOfType(
in oneOfType)
ATTRIBUTE(WSPost, WSPath = "/echoOneOfType")
RETURNS(oneOfType)
DEFINE out oneOfType
LET out = in
DISPLAY out._selector
CASE
WHEN out._selector == 2
DISPLAY "This is the schema 1 with id required property: ",
out.accountById.id
WHEN out._selector == 3
DISPLAY "This is the schema 2 with STRING value: ",
out.accountByName.name
WHEN out._selector == 4
DISPLAY "This is the schema 3 with DATE value: ",
out.accountBydate.date
END CASE
RETURN out
END FUNCTION
In the OpenAPI documentation, the GWS creates the JSON schema with oneOf
and
AdditionalProperties
properties set to false.
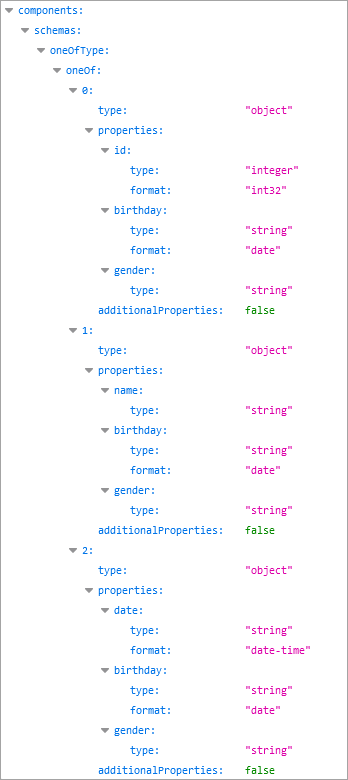